Build instant-sync distributed applications
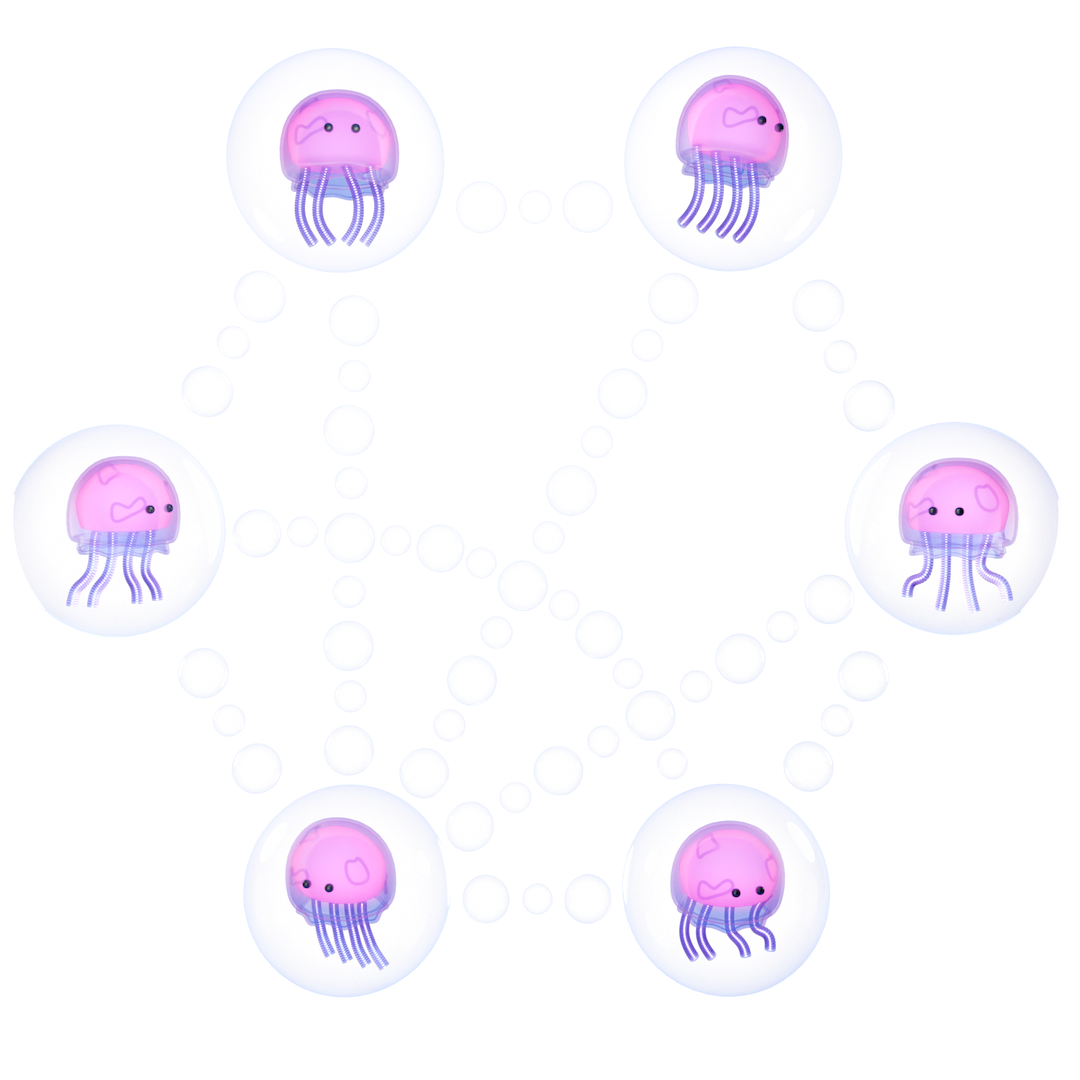
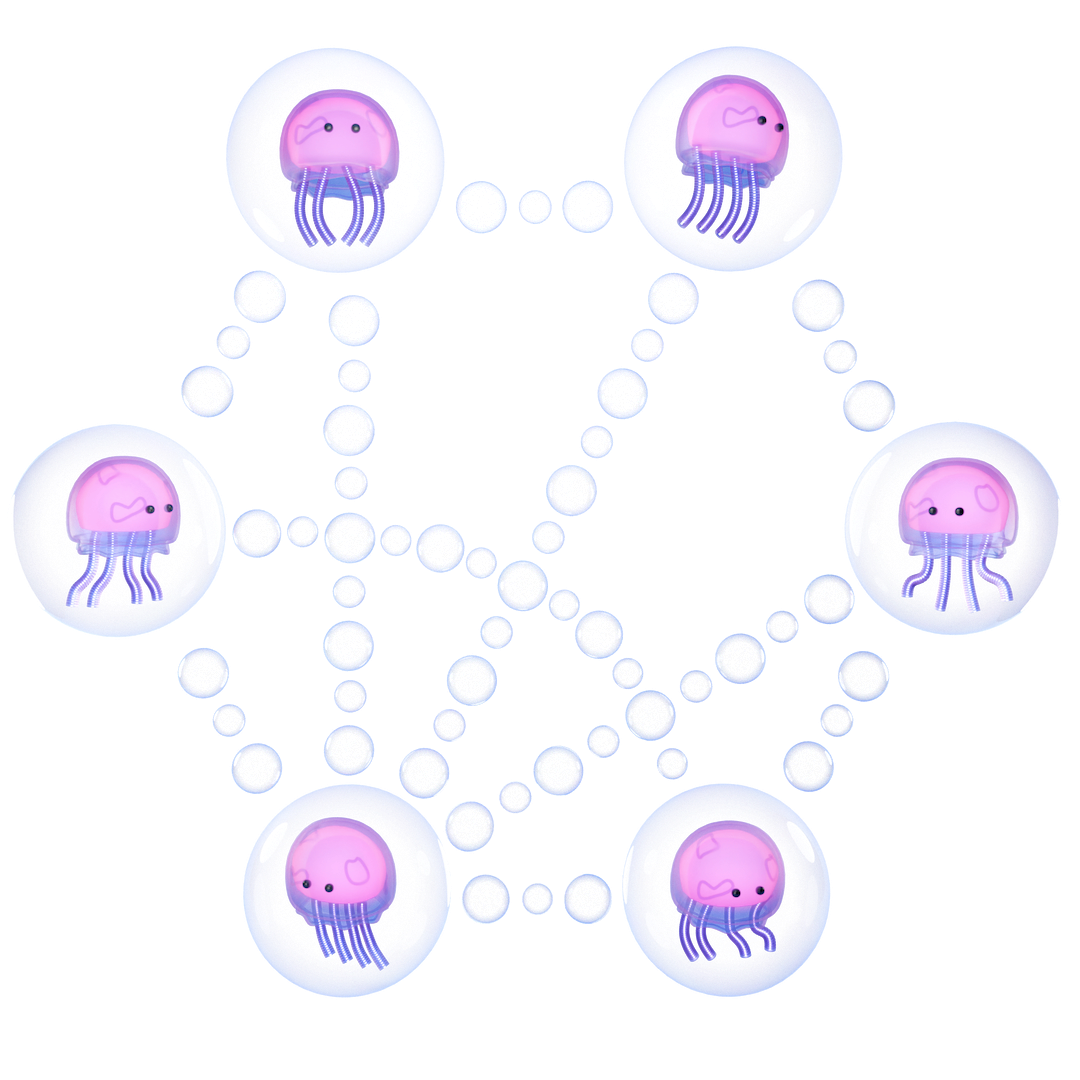
Canvas is an open-source database, like a decentralized, verifiable version of Firebase.
Write your application logic inside the database, and it syncs instantly with peers.
Users can access the database concurrently, and interactions are automatically merged, without central servers.
Use it to build mini-apps, multiplayer games, protocols, and decentralized networks, using the languages you already know.
Quick start
import { Canvas, Contract, ModelSchema } from "@canvas-js/core"
const Chat = {
models:
messages: {
id: "primary",
content: "string",
address: "string",
$rules: {
create: "address === this.address",
update: false,
delete: false,
}
}
} satisfies ModelSchema
}
const app = await Canvas.initialize({
topic: "example.xyz",
contract: Chat,
})
app.create("messages", {
content: "Hello world!",
address: this.address
})
import { Canvas, Contract, ModelSchema } from "@canvas-js/core"
class Chat extends Contract<typeof Chat.models> {
static models = {
messages: {
id: "primary",
content: "string",
address: "string",
}
} satisfies ModelSchema
async createMessage(content: string) {
this.db.create("messages", {
content,
address: this.address
})
}
}
const app = await Canvas.initialize({
topic: "example.xyz",
contract: Chat,
})
app.actions.createMessage("Hello world!")
import { useCanvas, useLiveQuery } from "@canvas-js/hooks"
import { Chat } from "./contract.ts"
const wsURL = process.env.SERVER_WSURL || null
export const App = () => {
const { app, ws } = useCanvas(wsURL, {
topic: "example.xyz",
contract: Chat,
})
const items = useLiveQuery(app, "messages")
return (<div>
<ComposeBox
onSend={app.actions.createMessage}
/>
<ItemView content={items}></ItemView>
</div>)
}
Every application is defined as a Canvas
class.
For simple applications, you can create a database-style Contract, where your backend logic goes in $rules
.
For complex applications, you can create a Class Contract, that lets you define custom actions and mutators.
Once you have a contract, you can run it from the command line:
canvas run contract.ts --topic example.xyz
[canvas] Bundled .ts contract: 4386 chars
[canvas] Serving HTTP API: ...
This starts a peer that you can connect to from your browser. By default, it will also connect to other servers on the application's topic.
Each contract can run inside the browser (using IndexedDB), on your desktop (using SQLite), or on servers (using SQLite/Postgres).
Read on to learn how to authenticate users, upgrade your app, or deploy to a server.
About Canvas
Canvas is based on several years of research on a new architecture for distributed web applications. It builds on work from projects including IPFS, OrbitDB, and other peer-to-peer databases.
We've published some of our technical presentations here:
- Merklizing the Key/Value Store for Fun and Profit
- Introduction to Causal Logs
- GossipLog: Reliable Causal Broadcast for libp2p
- GossipLog: libp2p Day Presentation
The current release of Canvas is an early developer preview that we are using in a limited set of production pilots. This first release is particularly recommended for mini apps and protocolized applications with public data, that anyone can permissionlessly interoperate with.
In 2025, we are working to expand the categories of applications that we can support, and provide support to developers building on the system. For more information, please reach out on Discord.